GitHub Actions
Automate, customize, and execute your software development workflows right in your repository with GitHub Actions. You can discover, create, and share actions to perform any job you'd like, including CI/CD, and combine actions in a completely customized workflow.
Prepare Docker Hub repository
Create a repository in your Docker hub for this GitHub repository Docker image
Create workflow in GitHub Actions
Go to the Actions page of your GitHub repository, and create a whatever workflow you'd like.
Write workflow and define env
Use a branch dev to trigger the CI. Define the build args "PROFILE" or any other argument in the workflow and you will use it as the profile to run SpringBoot. Be noted that you should define the Docker Hub account and repository here.
on:
push:
branches:
- dev
jobs:
hello_world_job:
runs-on: ubuntu-latest
name: A job to build and push docker
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v1
- name: Login to DockerHub
uses: docker/login-action@v1
with:
username: ${{ secrets.DOCKER_HUB_USER }}
password: ${{ secrets.DOCKER_HUB_PASS }}
- name: Build and push
uses: docker/build-push-action@v2
with:
context: .
file: ./Dockerfile
pull: true
push: true
cache-from: type=registry,ref=hustakin/hello-world-docker-action:latest
cache-to: type=inline
tags: hustakin/hello-world-docker-action:latest
build-args: PROFILE=nectar,ARG2=test
Create secrets for the Github repository
You should create the secrets DOCKER_HUB_USER (Docker Hub account) and DOCKER_HUB_PASS (Docker Hub password) as per you defined in the above workflow.
Create Dockerfile
Create a Dockerfile in the root folder of your project. You can specify the build argument in the Dockerfile and read it. You will see the Github Action workflow triggered after you push the code to the dev branch.
See GitHub Actions workflow logs
You will see the build argument PROFILE could be get in your Dockerfile.
Write Dockerfile for SpringBoot
The jar file of your SpringBoot project can be built by command: mvn clean package -Dmaven.test.skip=true. Write the Dockerfile to run your SpringBoot jar file. Please be noted you cannot read the ARG directly, but you can pass it to an env variable and read the variable instead.
FROM openjdk:8-jre
ARG PROFILE
ENV PROFILE_VAR=$PROFILE
VOLUME /tmp
# Add the built jar for docker image building
ADD target/hello-world-docker-action.jar hello-world-docker-action.jar
ENTRYPOINT ["/bin/bash", "-c", "java","-Dspring.profiles.active=$PROFILE_VAR","-jar","/hello-world-docker-action.jar"]
# DO NOT USE(The variable would not be substituted): ENTRYPOINT ["java","-Dspring.profiles.active=$PROFILE_VAR","-jar","/hello-world-docker-action.jar"]
# CAN ALSO USE: ENTRYPOINT java -Dspring.profiles.active=$PROFILE_VAR -jar /hello-world-docker-action.jar
EXPOSE 80
Another way to pass variables
There is another way to pass variables if the above way doesn't work. You can put the command into a script file and add the shell as entrypoint.
FROM openjdk:8-jre
ARG PROFILE
ENV PROFILE_VAR=$PROFILE
VOLUME /tmp
# Add the built jar for docker image building
ADD target/hello-world-docker-action.jar hello-world-docker-action.jar
# Build a shell script because the ENTRYPOINT command doesn't like using ENV
RUN echo "#!/bin/bash \n java -Dspring.profiles.active=${PROFILE_VAR} -jar /hello-world-docker-action.jar" > ./entrypoint.sh
RUN chmod +x ./entrypoint.sh
# Run the generated shell script.
ENTRYPOINT ["./entrypoint.sh"]
EXPOSE 80
Write workflow to build jar
Add a step to run the maven building command in order to get the above jar file.
on:
push:
branches:
- dev
jobs:
hello_world_job:
runs-on: ubuntu-latest
name: A job to build and push docker
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up JDK 1.8
uses: actions/setup-java@v1
with:
java-version: 1.8
- name: Build with Maven
run: mvn clean package -Dmaven.test.skip=true
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v1
- name: Login to DockerHub
uses: docker/login-action@v1
with:
username: ${{ secrets.DOCKER_HUB_USER }}
password: ${{ secrets.DOCKER_HUB_PASS }}
- name: Build and push
uses: docker/build-push-action@v2
with:
context: .
file: ./Dockerfile
pull: true
push: true
cache-from: type=registry,ref=hustakin/hello-world-docker-action:latest
cache-to: type=inline
tags: hustakin/hello-world-docker-action:latest
build-args: PROFILE=nectar,ARG2=test
Add more workflow for other branches
Add another branch for another environment. Each workflow yml -> Specific trigger branch -> Specify each profile value for the env -> Run the specific profile SpringBoot application.
Take another trigger branch and env "amazon" for example:
Manually/Automatically run docker container creating scripts
In development mode, when you want to build a specific env you could push the code to the related trigger branch to let workflow run. After the workflow finish, you can manually run a shell script in the specific env to build docker container from the latest docker image in Docker Hub.
You could also add a WebHook in your workflow or in your Docker Hub to trigger the automatic building scripts for specific env.
Angular profiles passing
Regarding Angular project, you will need to add env profiles in the "configurations" node of the angular.json, and add commands of different envs in your package.json. Now you could create workflows for all your env and execute different npm command to build.
In your Angular app, you could import the environment and read the settings inside it.
Add a workflow status badge
You can display a status badge in your repository to indicate the status of your workflows. A status badge shows whether a workflow is currently failing or passing. A common place to add a status badge is in the README.md file of your repository, but you can add it to any web page you'd like. By default, badges display the status of your default branch. You can also display the status of a workflow run for a specific branch or event using the branch and event query parameters in the URL.

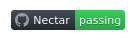